この記事では、1つの画面に複数のUICollectionView
を並べる方法を紹介します。UICollectionView
の使い方については、以下の記事をみてみてください。
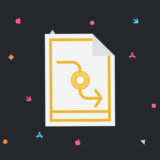
やりたいこと
まず、やりたいこととしては、1つの画面に複数のUICollectionView
を配置することです。
このようなイメージです。
実装方法
実装方法はそれほど変わるところはありません。セルを作るときやセルをタップしたときに、処理を分岐させるというイメージです。
処理を分岐させるので、どちらのCollectionView
かを区別する必要があります。区別する方法としては、Tag
の方法と名前で区別する方法の2パターンあります。
collectionView.tagで区別する方法
まずは、CollectionView
にそれぞれTag
をつけてください。
そしたら、あとはそのTag
で区別するだけです。if文でもSwitch文でもOKです。
extension ViewController: UICollectionViewDelegate, UICollectionViewDataSource { func numberOfSections(in collectionView: UICollectionView) -> Int { if collectionView.tag == 0 { return 1 } else { return 1 } } func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int { if collectionView.tag == 0 { return 10 } else { return 20 } } func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell { if collectionView.tag == 0 { let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "Cell1", for: indexPath) return cell } else { let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "Cell2", for: indexPath) return cell } } }
名前で区別する方法
それぞれ@IBOutlet
で紐づけます。
@IBOutlet weak var firstCollectioView: UICollectionView! @IBOutlet weak var secondCollectionView: UICollectionView!
あとは、パターン1のtagと同じくif文やSwitch文で分岐させるだけです。
extension ViewController: UICollectionViewDelegate, UICollectionViewDataSource { func numberOfSections(in collectionView: UICollectionView) -> Int { if collectionView == firstCollectioView { return 1 } else { return 1 } } func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int { if collectionView == firstCollectioView { return 10 } else { return 20 } } func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell { if collectionView == firstCollectioView { let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "Cell1", for: indexPath) return cell } else { let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "Cell2", for: indexPath) return cell } } }
参考文献