この記事では、Firebaseを使ってGoogleのログイン機能を簡単に実装していきたいと思います。
こんな感じのログイン機能が作れます。
実装方法
Firebaseの登録を行なっていない方は、以下の記事を参考にプロジェクトを登録してください。
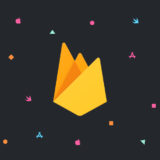
まずは、Firebaseの方でGoogleログインを許可します。
①Authentivationをクリック
②始めるをクリック
Googleをクリック
①有効にする
②サポートのメールアドレスを入力
③保存をクリック
CocoaPodsで以下のライブラリを追加でインストールしてください。
pod 'Firebase/Auth' pod 'GoogleSignIn'
CocoaPodsのやり方がわからない方は、こちら
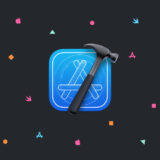
①GoogleService-Info.plistを選択
②REVERSED_CLIENT_IDのValueをコピー
①プロジェクトを選択
②TERGETSを選択
③Infoを選択
④プラスボタンをクリック
⑤URL Schemesにペースト
AppDelegate.swift
にimportしましょう。
import GoogleSignIn
AppDelegate.swift
に以下の関数を追記してください。
func application(_ application: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey: Any]) -> Bool { return GIDSignIn.sharedInstance.handle(url) }
分かりやすいように背景色を適当な色に変更しておきます。
①Viewをクリック
② (Show The Attributes inspector)をクリック
③Backgroundを適当な色に変更
Viewを適当な場所に配置
①Viewを選択
② (Show The Identifier inspector)をクリック
③ClassでGIDSigninButtonを選択
コードと2分割して紐付けましょう。
①NameにdidTapSoginButton
と入力
②EventをTouchUpInsideに変更
③Connectをクリック
ViewController.swift
にもimportしましょう。
import Firebase import GoogleSignIn
以下がログインのコードです。ViewController.swift
に貼り付けてください。
private func auth() { guard let clientID = FirebaseApp.app()?.options.clientID else { return } let config = GIDConfiguration(clientID: clientID) GIDSignIn.sharedInstance.signIn(with: config, presenting: self) { [unowned self] user, error in if let error = error { print("GIDSignInError: \(error.localizedDescription)") return } guard let authentication = user?.authentication, let idToken = authentication.idToken else { return } let credential = GoogleAuthProvider.credential(withIDToken: idToken, accessToken: authentication.accessToken) self.login(credential: credential) } } private func login(credential: AuthCredential) { print("ログイン完了") }
ボタンを押したときにauth()
を呼びましょう。
実行して確認しましょう。Googleでログインできるようになっているはずです。
これでFirebaseで簡単にログイン機能が作れました。
import UIKit import Firebase import GoogleSignIn @main class AppDelegate: UIResponder, UIApplicationDelegate { func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { FirebaseApp.configure() return true } // MARK: UISceneSession Lifecycle func application(_ application: UIApplication, configurationForConnecting connectingSceneSession: UISceneSession, options: UIScene.ConnectionOptions) -> UISceneConfiguration { // Called when a new scene session is being created. // Use this method to select a configuration to create the new scene with. return UISceneConfiguration(name: "Default Configuration", sessionRole: connectingSceneSession.role) } func application(_ application: UIApplication, didDiscardSceneSessions sceneSessions: Set<UISceneSession>) { // Called when the user discards a scene session. // If any sessions were discarded while the application was not running, this will be called shortly after application:didFinishLaunchingWithOptions. // Use this method to release any resources that were specific to the discarded scenes, as they will not return. } func application(_ application: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey: Any]) -> Bool { return GIDSignIn.sharedInstance.handle(url) } }
import UIKit import Firebase import GoogleSignIn class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view. } @IBAction func didTappSignInButton(_ sender: Any) { auth() } private func auth() { guard let clientID = FirebaseApp.app()?.options.clientID else { return } let config = GIDConfiguration(clientID: clientID) GIDSignIn.sharedInstance.signIn(with: config, presenting: self) { [unowned self] user, error in if let error = error { print("GIDSignInError: \(error.localizedDescription)") return } guard let authentication = user?.authentication, let idToken = authentication.idToken else { return } let credential = GoogleAuthProvider.credential(withIDToken: idToken, accessToken: authentication.accessToken) self.login(credential: credential) } } private func login(credential: AuthCredential) { print("ログイン完了") } }